7view
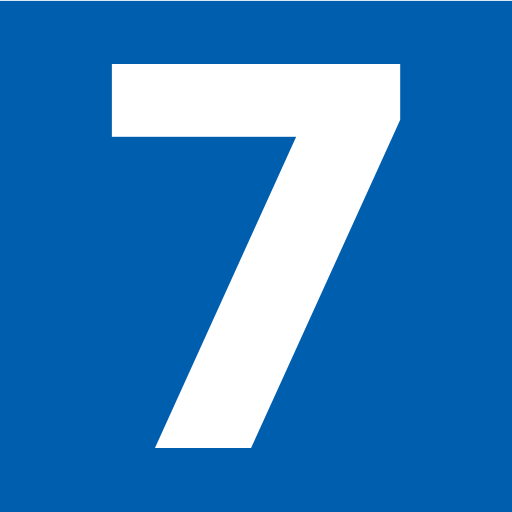
A cross-platform hl7 viewer for Windows, Linux und MacOS.
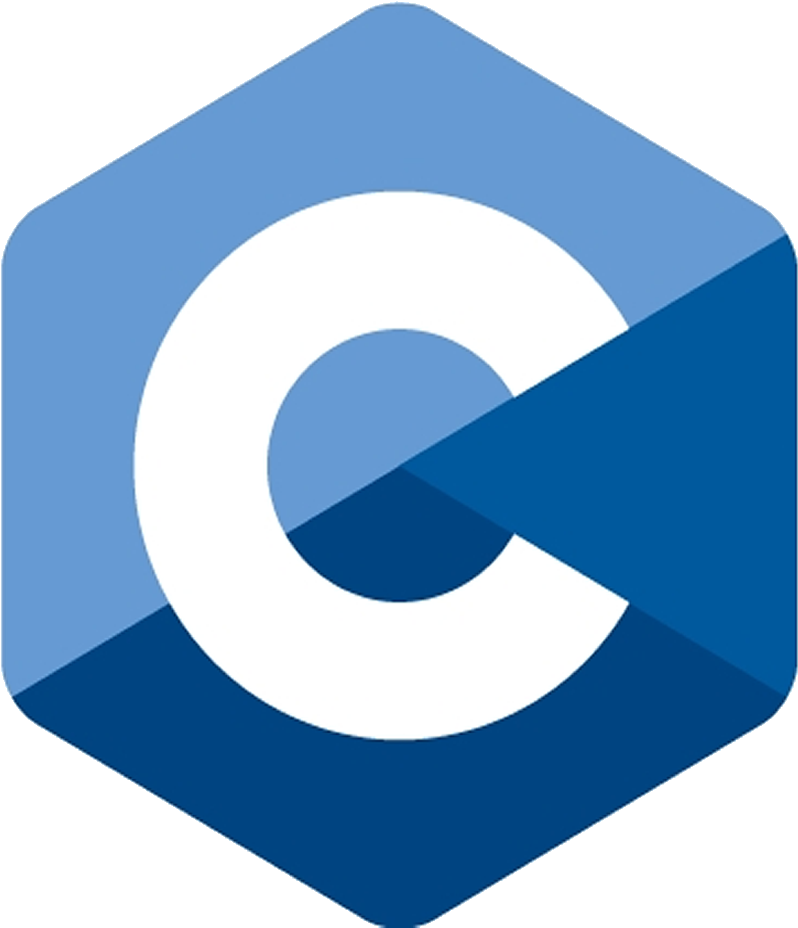
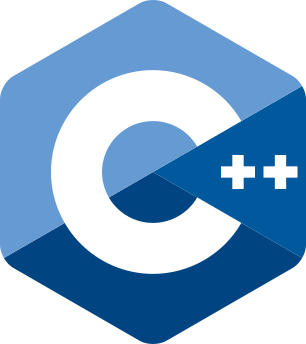
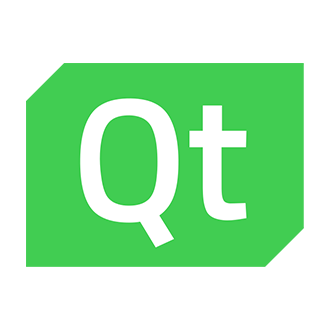
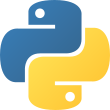
7View
Cross-platform HL7 viewer for Linux, MacOS and Windows
7view
is a simple and fast hl7 viewer that can be used on Windows, MacOS and Linux. The main features of the viewer are:
- Syntax highlighting: Delemiters and segment names are colorized
- Large file handling: can deal with large files such as Base64 embedded MDM messages
- Shell integration: for gui applications (Explorer and Finder context menu)
- Search: search for keywords in a file
- Base64 decode: fields and open them in external viewer
- HEX Viewer: for inspecting specific fields (usefull for analyzing encoding problems)
- All sources are freely available and licensed under the LGPL. Report issues on gitlab.com/wunderlins/hl7parse
This project also contains a collection of hl7 utilities written in C and C++. All tools are available and tested on Debian, Windows and MacOS. The packaged tools provide the following functionality:
- gui tools
- 7view - a gui viewer for HL7 files
- 7pdf - extract a base64 encoded string from a field and open it in a pdf viewer
- command line tools
- 7parse - create a command line grep-able tree representation of a hl7 file
- 7search - fast hl7 content search utility that knows the structure
- developer tools
- lib7 - the C implementation of the hl7 parser (API Documentation)
- pylib7 - python bindings for the C parser (API Documentation)
Download
System | Download | System Requirements |
---|---|---|
Windows | Installer, ZIP, Source | Windows 7/10 (maybe Vista, 64 bit only) |
MacOS | Disk Image (dmg), Source | MacOS 10.15 (Catalina) |
Debian | Debian Package (deb), Source | Debian Buster or equivalent Ubuntu |
Linux | x64 binary, Source | |
Python3 | pip package | Tested with Python 3.8+ (no python2 support) |
Disclaimer
All work product by the team is provided ββAS ISβ. Other than as provided in this agreement, the team makes no other warranties, express or implied, and hereby disclaims all implied warranties, including any warranty of merchantability and warranty of fitness for a particular purpose.
Release 0.4.1 - moar HEX’n streams
The 0.4.1 Release is a maintanance release. with a few new features.
- Open Base64 embedded documents in external viewers.
- Render files while they are parsed line by line, this is useful for very large files
- Progress bar while loading, also useful for very large files
- HEX viewer for inspecting binary content in a field.
- Shell integration on windows (when installer is used).
- Platform specific installers (deb, exe, dmg).
A lot of infrastructure has been changed
- gmake to cmake migration, better IDE integration and better crossplatform capabilities
- doxygen & sphinx for api documentation
- installers for all 3 supported platforms
- python setuptools package
- unit-tests for lib7 with ctest and a little bit for 7view with qtest
- experimental node editing functionality
- added CI, gitlab will now compile every commit to master
- with camke: better integration into IDEs such as Qt Creator, VS Code on linux, macos and windows (with mingw)
- added code of conduct
- added sub-project QHexView (made some for macos font rendering, assimilated into project tree)
- new icon / logo
- python lib now implemented with ctypes
- hl7_meta_t is now a member of message_t. Hl7 metadata is now always part of a message object.
- better error handling in viewer
- lib7 has gotten some callbacks for progress/start/end and when a new segment is available
- 7view will render line by line
- 7view loads the file in separate thread and shows a progress bar
Source Code
1me@there:~$ git clone https://gitlab.com/wunderlins/hl7parse.git
2me@there:~$ git submodule init # optional for doxygen theme
3me@there:~$ git submodule update # optional for doxygen theme
Python module
Install
1pip install --user lib7-X.X.X.tar.gz
Uninstall
1pip uninstall -y "$(pip freeze --user | grep lib7)"
Usage
Example from the API Documentation:
1import lib7
2
3msg = lib7.open("path/to/some/file.hl7")
4
5# check what sort of message type this is, defined in MsH-9.1
6msh91 = None
7msh92 = None
8try:
9 msh91 = msg.get("MSH-9.1")
10 print(msh91) # should print the content of the FIELD
11except Hl7StructureException:
12 print("MSH-9.1 Not found)
13
14try:
15 msh92 = msh91.next_sibling
16except Hl7StructureException:
17 print("MSH-9.2 Not found)
18
19root = msh92
20while root.parent:
21 root = root.parent
22
23# we have now found the top most node which is of type MESSAGE
24# This node contains N segments in children
25print(root.type) # prints: Type.MESSAGE
26print("%r" % root) # prints: <MESSAGE (children: 2)>
27print(root.num_children) # prints: 2
28assert(root == msg) # same same but different (variable name)
29
30# every node in the structure is an iterator which will
31# iterate over all child nodes.
32#
33# This is the preferred way, because it is faster than first
34# fetching all nodes via `n.children` and then looping over them.
35for seg in root:
36 print("%r" % seg)
37 # prints: <SEGMENT MSH(1) (children: 18), MSH>
38 # <SEGMENT PID(1) (children: 1), PID>
39
40 print(seg.addr)
41 # prints: MSH(1)
42 # PID(1)
43
44# or you may fetch a list of child ndoes like this:
45children = root.children
46
47# print the segment names
48for c in children:
49 print(c.data) # prints: MSH, PID, ...