#! shebang for C programs
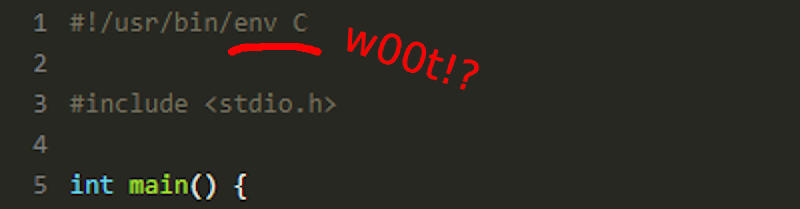
For testing it is sometimes very tedious to always compile and run the small C programs I use as utilities. So I thought, why not use the shebang for this. After investigating how the shebang mechanism works I figured a small bash script would do the trick.
The following script needs to be somewhere in your $PATH, I call the script “C”:
1#!/usr/bin/env bash
2
3filename=$(basename "$1")
4filename="${filename%.*}"
5
6# need to compile?
7if [ "$1" -nt "/tmp/runcache/$filename" ]; then
8 mkdir -p /tmp/runcache/
9 # get rid of shebang line in C program and compile it
10 tail -n +2 "$1" > /tmp/runcache/$filename.c
11 gcc -Wall -std=c99 $CC_OPTS -o \
12 /tmp/runcache/$filename \
13 /tmp/runcache/$filename.c
14fi
15
16# first parameter is the old executable,
17# remove it and point to the compiled executable
18shift
19
20/tmp/runcache/$filename "$@"
Once in place, you can add the shebang line !#/usr/bin/env C
to your C code in the first line, make it executable and run it like a script. Here is our test C program:
1#!/usr/bin/env C
2
3#include <stdio.h>
4
5int main() {
6 printf("Hello World\n");
7 return 0;
8}
Then run it like this:
1$ chmod 700 test.c
2$ ./test.c
3Hello World
4$